Monitoring Data Exports in MS Dynamics CRM
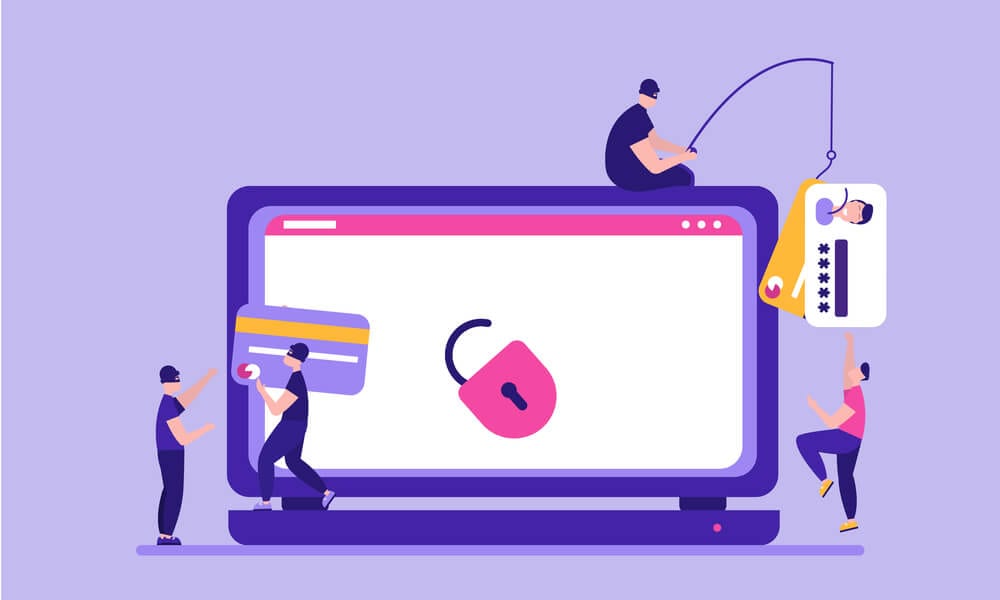
Monitoring data exports from Microsoft Dynamics CRM on-premises involves tracking user activities and system events to identify instances of data export. While the specifics can vary based on your organization's setup and security policies, here are some general steps you can take to monitor data exports:
1. Audit Logging: Dynamics CRM provides audit logging capabilities that allow you to track user actions, including data exports. You can enable auditing for specific entities or fields that you want to monitor. When auditing is enabled, CRM records details about changes made to the tracked entities, including export activities. Audit logs can be accessed and analyzed by administrators.
2. Security Roles and Privileges: Ensure that user roles and privileges are properly configured. Limit data export permissions to only those users who need them for their roles. By controlling access, you reduce the number of users who can perform exports.
3. User Access Logs: Monitor user access logs for the Dynamics CRM system. This can include tracking user logins, logouts, and session durations. Unusual login times or unexpected access patterns might indicate suspicious behavior.
4. Integration with SIEM Solutions: Consider integrating your Dynamics CRM system with Security Information and Event Management (SIEM) solutions. These solutions can aggregate and analyze logs from various sources, providing insights into user activities, including data exports.
5. Custom Logging and Alerts: Implement custom logging mechanisms within your CRM system to capture specific events, including data exports. You can set up alerts or notifications to be triggered when certain actions are performed, allowing administrators to be alerted in real-time.
6. Network and Firewall Monitoring: Monitor network traffic and firewall logs to identify large data transfers or unusual patterns that could indicate data exports. This can help you detect potential unauthorized data exports.
7. Database Activity Monitoring: Implement database activity monitoring tools to track database-level activities, including queries and exports. This can help you identify direct queries made to the database that might not be captured by CRM's built-in auditing.
8. User Training and Awareness: Educate users about the importance of data security and the proper procedures for exporting data. By raising awareness, you encourage responsible data handling practices.
9. Regular Audits: Conduct regular audits of data export activities to identify any discrepancies or unauthorized activities. Compare the audit logs with user-reported activities to ensure they align.
10. Incident Response Plan: Have a well-defined incident response plan in place. If you identify suspicious or unauthorized data export activities, you should be prepared to take appropriate actions to mitigate the risk.
Remember that data privacy and security regulations (such as GDPR, HIPAA, etc.) may impact how you monitor and handle data export activities. Always ensure that your monitoring practices comply with relevant regulations and best practices.
Implementing a comprehensive data export monitoring strategy involves a combination of technical solutions, policies, and user awareness. It's a good idea to work closely with your IT security team and CRM administrators to design and implement an effective monitoring approach tailored to your organization's needs.
using System;
using Microsoft.Xrm.Sdk;
using Microsoft.Xrm.Sdk.Query;
namespace DataExportLoggingPlugin
{
public class DataExportLoggingPlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
// Obtain the execution context and organization service
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
IOrganizationServiceFactory serviceFactory = (IOrganizationServiceFactory)serviceProvider.GetService(typeof(IOrganizationServiceFactory));
IOrganizationService service = serviceFactory.CreateOrganizationService(context.UserId);
try
{
// Check if the plugin is triggered on the "Export" action
if (context.MessageName == "Export" && context.PrimaryEntityName == "systemuser")
{
// Extract necessary data from the context
Guid userId = context.UserId;
string userName = context.InitiatingUserId.ToString();
DateTime exportTime = DateTime.Now;
// Log the primary entity name and view name
string primaryEntityName = context.PrimaryEntityName;
string viewName = context.Parameters.Contains("ViewName")
? context.Parameters["ViewName"].ToString()
: "N/A";
// Create a log record entity
Entity logRecord = new Entity("custom_exportlog");
logRecord["custom_userid"] = new EntityReference("systemuser", userId);
logRecord["custom_exporttime"] = exportTime;
logRecord["custom_exportdetails"] = $"Data export performed by user: {userName}. Primary Entity: {primaryEntityName}, View Name: {viewName}";
// Create the log record
service.Create(logRecord);
}
}
catch (Exception ex)
{
// Handle exceptions and log errors appropriately
throw new InvalidPluginExecutionException("An error occurred in the DataExportLoggingPlugin: " + ex.Message, ex);
}
}
}
}
In this example, the plugin is triggered on the "Export" action and captures the export event for the "systemuser" entity. It creates a custom entity named "custom_exportlog" to store the export event details, including the user who performed the export and the export time. Make sure to create this custom entity and fields before deploying the plugin.
Configure the Plugin Step:
- Click on "New" to add a new Component of type "Plug-in Step."
- Configure the Plugin Step as follows:
- Message:
Export
- Primary Entity:
systemuser
- Event Pipeline Stage: Choose the appropriate stage based on your requirements. In this case, you might choose
PreOperation
. - Execution Mode: Choose
Synchronous
. - Deployment: Choose the appropriate deployment based on your needs.
- Filter Criteria: Define any filter criteria if needed.
- Impersonation: Choose the appropriate option based on security requirements.
- Plugin: Choose the plugin type you defined earlier.
- Configuration: Leave this empty or configure it based on your plugin's requirements.
- Message:
The choice of the event pipeline stage depends on your requirements. In the example I provided earlier, the code was written to execute in the PreOperation
stage. This stage allows you to capture information before the actual export operation takes place. Depending on your use case, you might choose a different stage.